Pipl Thumbnails API
As an API subscriber, you also enjoy complimentary access to our Thumbnail API. The Thumbnail API saves you the hassle of dealing with profile pictures by providing built-in features for:
- Cropping images to the right dimensions
- Zooming in on faces with advanced face detection
- Attributing sources by adding favicons to images
- Image redundancy—specify a fallback image
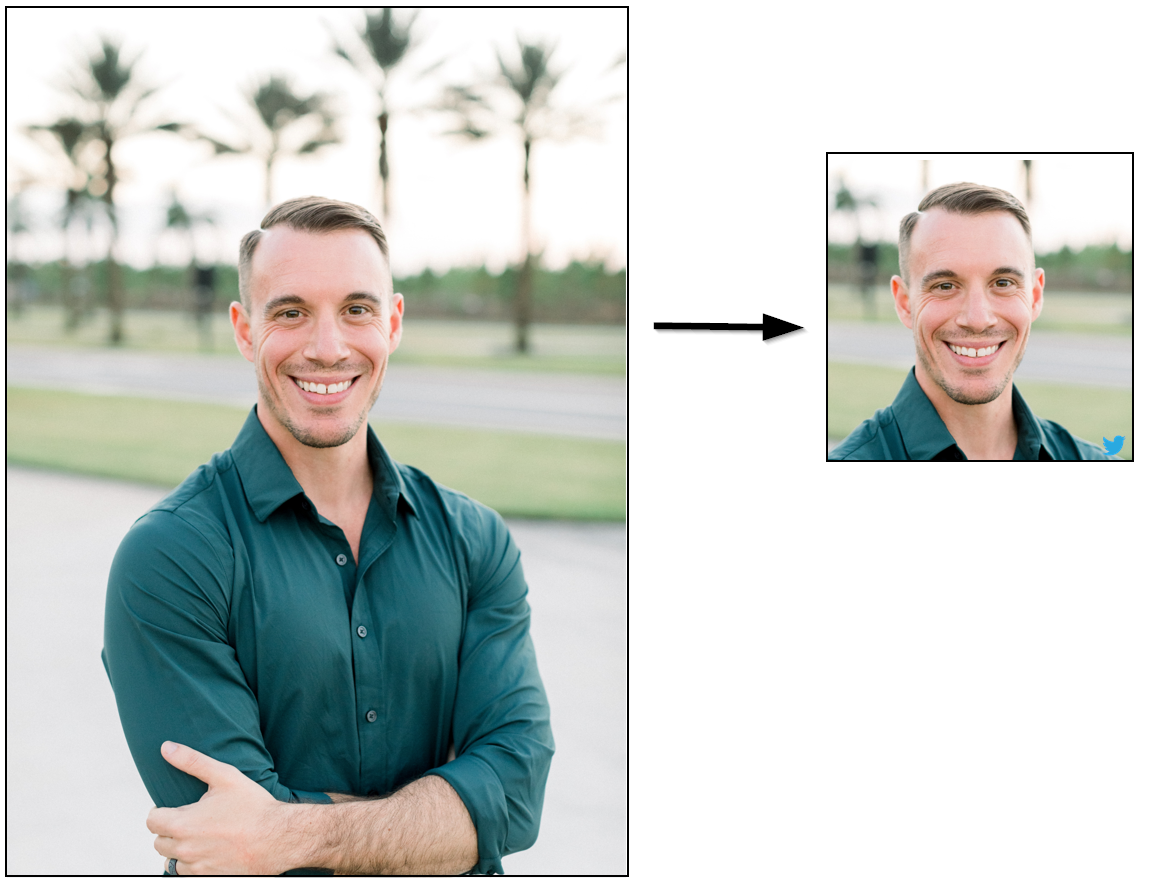
How does it work?
Each image field in our API responses includes a special token that can be sent to our thumbnail servers. You can specify the exact parameters depending on your needs.
{
"url": "http://vignette3.wikia.nocookie.net/smallville/images/5/55/S10E18-Booster21.jpg",
"thumbnail_token": "AE2861B242686E7BD0CB4D9049298EB7D18FEF66D950E8AB78BCD3F484345CE74536C19A85D0BA3D32DC9E7D1878CD4D341254E7AD129255C6983E6E154C4530A0DAAF665EA325FC0206F8B1D7E0B6B7AD9EBF71FCF610D57D"
}
Creating a thumbnail URL
The base URL of our thumbnail server is: https://thumb.pipl.com/image. An example of a complete url would be:
http://thumb.pipl.com/image?height=250&width=250&favicon=true&zoom_face=true&tokens=AE2861B242686E7BD0CB4D9049298EB7D18FEF66D950E8AB78BCD3F484345CE74536C19A85D0BA3D32DC9E7D1878CD4D341254E7AD129255C6983E6E154C4530A0DAAF665EA325FC0206F8B1D7E0B6B7AD9EBF71FCF610D57D
A redundant thumbnail URL
Sometimes, images will no longer be available on the web. To help you create robust thumbnail URLs, our thumbnail API allows specifying a fallback token.
To use this option, send two tokens to the API separated by a comma, like this:
http://thumb.pipl.com/image?height=250&width=250&favicon=true&zoom_face=true&tokens=FIRST_TOKEN,SECOND_TOKEN
Allowed parameters:
Parameter | Description |
---|---|
tokens required | Required. One or two thumbnail tokens, separated by a comma. If the image referenced by the first thumbnail token is no longer available on the web, the second token will be used. |
height required | Height in pixels. Integer between 100-500. |
width required | Width in pixels. Integer between 100-500. |
favicon | Boolean. Whether to show favicon. |
zoom_face | Boolean. Whether to enable face zoom. |
Using the client libraries
If you use our client libraries, we’ll handle all of the boilerplate for you.
# Creating a thumbnail URL
thumbnail_url = image.get_thumbnail_url(200, 100, zoom_face=True, favicon=True)
# Creating a redundant image URL
redundant_url = Image.generate_redundant_thumbnail_url(first_image, fallback_image, 100, 100)
# Creating a thumbnail URL
thumbnail_url = image.thumbnail_url width: 200, height: 100, favicon: true, zoom_face: true
# Creating a redundant image URL
image = Pipl::Image.new(thumbnail_token: 'TOKEN')
fallback = Pipl::Image.new(thumbnail_token: 'FALLBACK_TOKEN')
url = image.thumbnail_url width: 200, height: 100, favicon: true, zoom_face: true, fallback: fallback
# Creating a thumbnail URL
String thumbUrl = image.getThumbnailUrl(200, 100, true, true, false);
# Creating a redundant image URL
String url = Image.generateRedundantThumbnailUrl(250, 250, true, true, false, resp.person.images.get(0), resp.person.images.get(1));
# Creating a thumbnail URL
$thumbUrl = $image->get_thumbnail_url(200, 100, true, true);
# Creating a redundant image URL
$thumbUrl = PiplApi_Image::generate_redundant_thumbnail_url($first_image, $second_image, 100, 100);
# Creating a thumbnail image URL
var thumbUrl = _image.GetThumbnailUrl(200, 100, true, true);
# Creating a redundant image URL
var thumbnail_url = Pipl.APIs.Data.Fields.Image.GenerateRedundantThumbnailUrl(image1, image2, 200, 100, true,true,true);
Special cases
In some cases, both of your tokens will reference an image no longer available online, or they might contain a default placeholder image and not a real image of the person.
In those cases, the thumbnail server will return 404.
Updated about 3 years ago